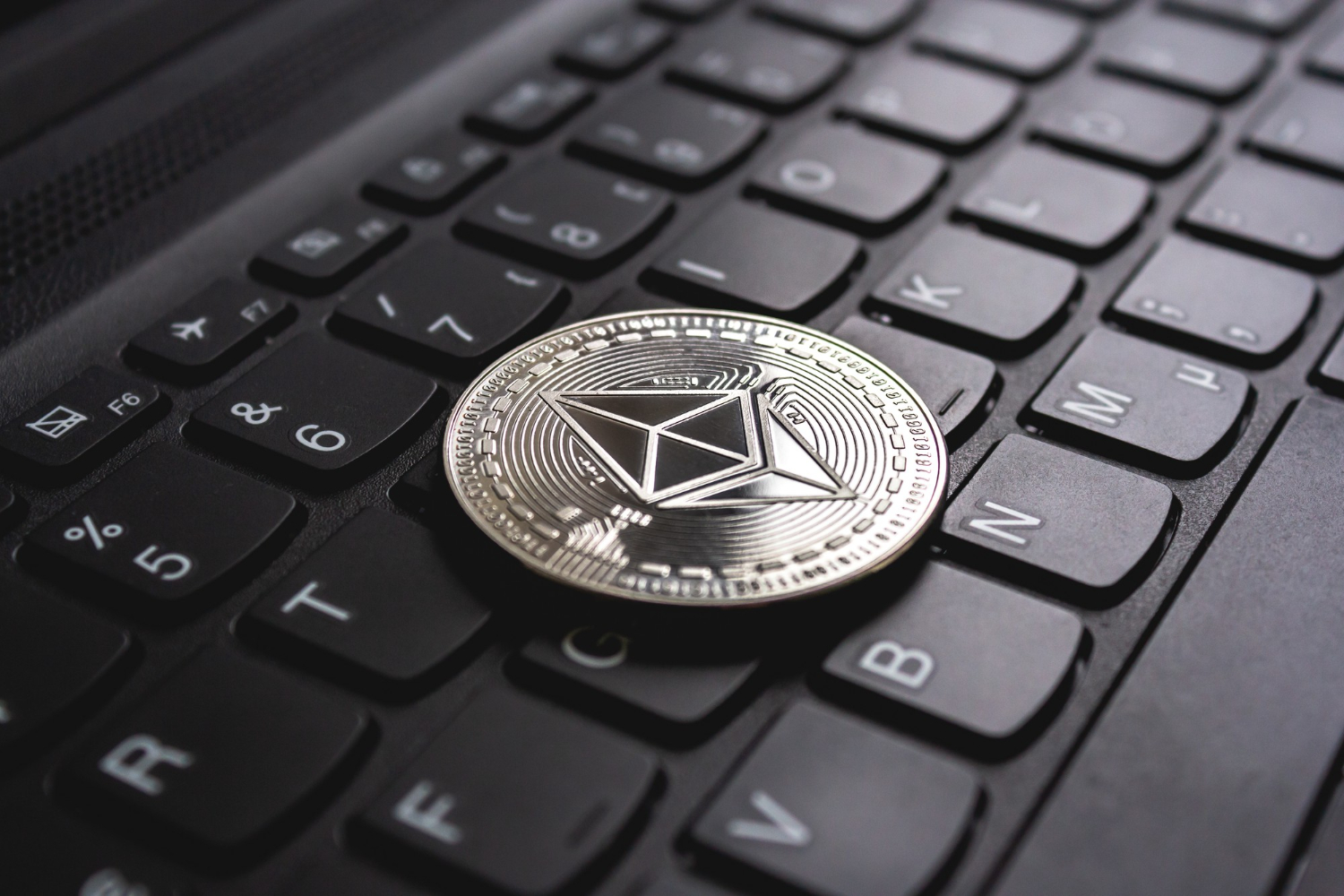
Smart contracts have become a fundamental building block of decentralized applications (DApps) on blockchain platforms like Ethereum. After smart contract development, rigorous testing is essential to ensure the security and reliability of these contracts. In this blog post, we will learn about the tools and how to test a smart contract.
Ethereum Smart Contract Testing
It's critical to comprehend the several testing methodologies, as well as the frameworks and tools used to test Ethereum smart contracts, before beginning any testing.
Types of Testing for Solidity Smart Contracts
There are several types of testing for Solidity smart contracts, each serving a specific purpose:
- Unit Testing: This involves testing individual functions or methods within a contract. It is the most detailed testing level and aids in finding problems at the code level.
- Integration Testing: Integration testing focuses on how different parts of the smart contract interact with each other. It ensures that the contract’s components work together harmoniously.
- Functional Testing: Functional tests verify that the contract behaves as expected from an end-user perspective. It tests whether the contract correctly executes its intended functionality.
- Security Audits: Security audits, often performed by external experts, identify vulnerabilities, including those that could lead to hacks or exploits.
- Gas Usage Testing: Testing the gas usage of your contract helps optimize its efficiency and minimize transaction costs for users.
Tools and Framework
Several tools and frameworks are available to help test Solidity smart contracts:
- Truffle: Truffle is a widely used development and testing framework for Ethereum. It provides various tools to compile, test, and deploy smart contracts.
- Hardhat: An Ethereum development environment with a testing framework is called Hardhat. For writing tests and administering them locally, it provides a wealth of support.
- Remix: Remix is an in-browser development and testing tool for Ethereum smart contracts. It is an excellent choice for quick contract testing and debugging.
Suggested Post | Analyzing Solidity and Vyper for Smart Contracts Programming
Test Case for an ERC-20 Smart Contract
In this part, we’ll use the Hardhat environment to test an ERC20 smart contract. The test scenarios for a typical ERC20 smart contract are listed below:
The “beforeEach” function deploys a new smart contract in the test environment for every “it” block in the test cases. Each “it” block has a description that denotes the functionality that the block tests.
const { expect } = require('chai'); const { ethers } = require('hardhat'); describe('Token contract', function () { let Token; let token; let owner; let buyer; beforeEach(async function () { Token = await ethers.getContractFactory('MyToken'); token = await Token.deploy(1000); [owner, buyer] = await ethers.getSigners(); }); describe('Deployment', function () { it('Should return the right owner as set during deployment', async function () { expect(await token.balanceOf(owner.address)).to.equal(1000); }); it('Should return the total supply as set during deployment', async function () { expect(await token.totalSupply()).to.equal(1000); }); }); describe('Transactions', function () { it('Should transfer tokens between different accounts', async function () { await token.transfer(buyer.address, 100); expect(await token.balanceOf(owner.address)).to.equal(900); expect(await token.balanceOf(buyer.address)).to.equal(100); }); it('Should fail if sender doesn’t have enough tokens for transfer', async function () { const initialOwnerBalance = await token.balanceOf(owner.address); await expect( token.transfer(buyer.address, 10000) ).to.be.revertedWithoutReason(); expect(await token.balanceOf(owner.address)).to.equal(initialOwnerBalance); }); it('Should update allowance after approve', async function () { await token.approve(buyer.address, 100); expect(await token.allowance(owner.address, buyer.address)).to.equal(100); }); it('Should transfer tokens from one account to another with allowance', async function () { await token.approve(buyer.address, 100); await token.transferFrom(owner.address, buyer.address, 100); expect(await token.balanceOf(owner.address)).to.equal(900); expect(await token.balanceOf(buyer.address)).to.equal(100); expect(await token.allowance(owner.address, buyer.address)).to.equal(0); }); it('Should fail if sender doesn’t have enough allowance', async function () { await token.approve(buyer.address, 99); await expect( token.transferFrom(owner.address, buyer.address, 100) ).to.be.revertedWith('ERC20: transfer amount exceeds allowance'); }); }); });
Also, Discover: A Definitive Guide to Smart Contract Development Tools
Smart Contract Development with Oodles
Programming languages that Oodles Blockchain's smart contract engineers are skilled in include Golang, Elixir, Solidity, and more. With our services for developing smart contracts, you may automate your business. Connect with our smart contract developers to discuss your project requirements.