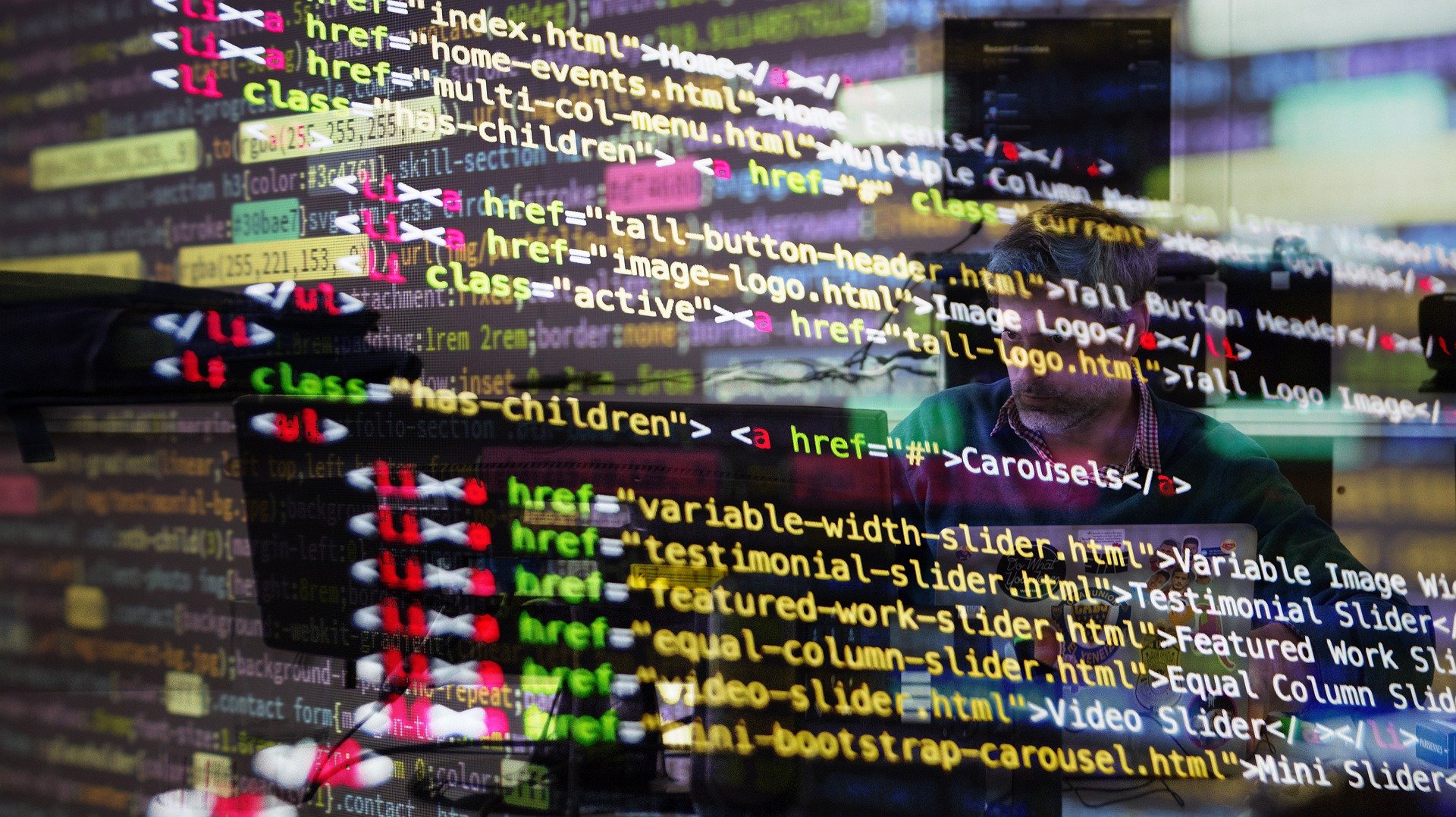
Modern programming relies on Python multithreading to unleash concurrent execution. Multithreading allows several execution threads in a single process, improving performance and responsiveness. Python's built-in `threading` module simplifies the creation and management of threads.
This blog post explores the meaning of multithreading in Python, its benefits, implementation method, and best practices. In addition, the article extends its reach to explain the diverse yet interesting world of encapsulation in Python, its uses, types, etc.
What is Multithreading?
Multithreading often means running numerous threads in one process. Threads let programs run several tasks simultaneously. Multithreading in Python helps programs handle real-time processing, parallel processing, and dynamic user interfaces.
Threads are lightweight execution units that allow programs to execute multiple operations simultaneously. Each thread has its instructions, program counter, and stack, but they share memory. This enables efficient communication and synchronization between threads.
An example of multithreading in Python using the 'threading' module:
import threading
# Define a function to be executed by the thread
def print_numbers():
for i in range(1, 6):
print("Thread 1:", i)
# Create a new thread
thread1 = threading.Thread(target=print_numbers)
# Start the thread
thread1.start()
# Main thread continues its execution
for i in range(1, 6):
print("Main Thread:", i)
# Wait for the thread to finish
thread1.join()
# The program execution completes
Explanation
In this example, you can create a new thread named 'thread' and designate the function 'print_numbers' as the thread's execution target. Using the 'start()' method, you can begin the thread. In the meantime, the main thread executes its own set of duties.
The primary thread and 'thread1' display the digits 1 through 5. Finally, you can use the 'join()' method to wait for 'thread1' to conclude before terminating the program. Due to the concurrent nature of threads, the output may differ, but it will demonstrate the concurrent execution of the main thread and 'thread1.'
How Does Multithreading Benefit Us?
Multithreading in Python has numerous advantages, including:
- PERFORMANCE-ORIENTED: Multithreading improves the overall performance of a program by conducting tasks concurrently, especially when dealing with computationally intensive or I/O-bound operations.
- HANDLES MULTIPLE TASKS: Multithread in Python enables programs to manage multiple tasks concurrently, making it suitable for applications requiring real-time processing, event processing, or parallel processing.
- CREATES RESPONSIVE USER-INTERFACES: Multithreading contributes to the maintenance of responsive user interfaces by offloading time-consuming operations to separate threads, preventing the primary thread from becoming blocked.
- EFFECTIVE RESOURCE USE: Multithreading improves the utilization of system resources, such as CPU processors, by distributing tasks efficiently across multiple threads.
Implementation of Multithreading in Python
Multithreading in Python can be achieved by following certain steps. There are many ways devised to execute this program; however, this article provides a step-by-step guide for multithreading using Python in a simple way. The steps follow:
- IMPORTING THE "THREADING" MODULE
This is the very first step. The "threading" module provides the necessary classes and functions for working with threads.
ILLUSTRATION: import threading (see above)
- DEFINING A FUNCTION OR CLASS
This is the second step. Create a function or class representing the task the thread will carry out. This function or class will serve as the thread's objective.
ILLUSTRATION:
def my_function():
# Code to be executed by the thread
# ...
- CREATING A NEW THREAD
Create a new 'Thread' object with the function or class defined in the preceding phase as the target.
ILLUSTRATION:
my_thread = threading.Thread(target=my_function)
- STARTING THE THREAD
Invoke the start() method on the thread object to initiate the thread's execution.
ILLUSTRATION:
my_thread.start()
- CONTINUING WITH THE MAIN THREAD
The main thread continues to execute its own set of duties concurrently with the new thread.
ILLUSTRATION:
# Code executed by the main thread
# ...
- WAITING FOR THE THREAD TO FINISH
Use the join() method on the thread object to wait for the thread to finish before exiting the program, if necessary.
ILLUSTRATION: my_thread.join()
Encapsulation in Python: What is it?
Encapsulation is a fundamental principle of object-oriented programming that organizes and safeguards data within objects. It enables aggregating related data and behaviors and controls their access and modification. The article will dissect encapsulation in Python, particularly.
Benefits of Encapsulation
Encapsulation serves its users a lot of benefits in the forms discussed below:
- PROTECTION OF DATA: Encapsulation enables the concealment of an object's internal implementation details and the protection of its data from unauthorized access and modification.
- ORGANIZATION OF CODE: Encapsulation facilitates code organization by combining related data and behaviors within objects. This increases the maintainability and legibility of the code.
- ABSTRACTION: Encapsulation provides an abstraction layer, enabling users of a class to interact with its public interface without being concerned with its internal implementation.
Encapsulation in Python: What does it Ensue?
Access modifiers can be used to implement encapsulation in Python. Although Python lacks built-in access modifiers like other programming languages (such as private and protected), it follows a convention of using underscores to denote the level of access:
- SINGLE UNDERSCORE ('_'): A variable or method is intended to be considered private. It is a convention and access to the variable or method from outside the class is still possible.
- DOUBLE UNDERSCORE ('__'): It triggers name mangling and effectively makes the variable or method private. It modifies the variable or method name to _ClassName__variable or _ClassName__method to prevent direct access outside the class.
WHAT ARE THE BEST PRACTICES FOR ENCAPSULATION IN PYTHON?
When working with multithreading and encapsulation in Python, the following best practices must be considered:
- THREAD SAFETY: To ensure correct synchronization and access control to shared resources to prevent race conditions and data corruption.
- GIL LIMITATION: Python has a Global Interpreter Lock (GIL) that precludes multiple threads from executing Python bytecodes simultaneously. This can limit the performance benefits of multithreading in Python for CPU-intensive operations.
- CONCURRENCY DESIGN: To consider task granularity, load balancing, and avoiding superfluous dependencies when designing multithreaded programs to maximize concurrency.
- COMMUNICATION AND DOCUMENTATION: To facilitate collaboration and code maintenance, document thread interactions, synchronization mechanisms, and shared resources in detail.
Conclusion
Multithreading in Python boosts program performance and responsiveness in programming and data science. Developers can build, manage, synchronize, and communicate threads using the threading module.
Python encapsulation helps organize and protect object data, making code modular and maintainable. These concepts and recommended practices can improve Python efficiency, thread safety, and code quality.