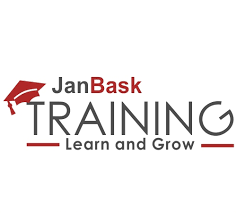
Algorithm problem solving techniques
When it comes to cracking a coding interview, having a strong foundation in algorithm problem solving techniques is crucial. In this article, we will explore some key techniques that can help you improve your problem-solving skills and increase your chances of success in data structure interview questions..
1. Understand the problem
Before jumping into solving a coding problem, it is essential to understand the problem statement thoroughly. Take your time to read and re-read the problem statement, identify the inputs and outputs, and clarify any doubts you may have. Understanding the problem clearly will help you come up with an appropriate algorithm.
2. Break down the problem
Breaking down the problem into smaller sub-problems is an effective strategy to tackle complex coding problems. Identify the core elements or sub-tasks required to solve the problem and develop a step-by-step approach. This approach will not only make the problem more manageable but also allow you to solve it more efficiently.
3. Choose appropriate data structures
Data structures play a crucial role in solving coding problems efficiently. Depending on the problem's requirements and constraints, choose the most appropriate data structure, such as arrays, linked lists, stacks, queues, trees, or graphs. Understanding the strengths and weaknesses of different data structures will enable you to select the optimal one for the given problem.
4. Implement the algorithm
Once you have developed the algorithm based on the problem analysis, it is time to implement it in code. Pay attention to code correctness, efficiency, and readability. Use appropriate variable names, follow coding best practices, and write modular code. Additionally, consider edge cases and handle exceptions gracefully.
5. Test and debug
After implementing the algorithm, thoroughly test it with various test cases to ensure its correctness. Test it with both the sample test cases provided in the problem statement and additional test cases that you can come up with. If any bugs or logic errors are encountered, debug them patiently and make the necessary corrections.
6. Analyze the time and space complexity
Understanding the time and space complexity of an algorithm is crucial for evaluating its efficiency. Analyze how the algorithm's time complexity scales with the input size and how much space it requires for execution. This understanding will help you evaluate the algorithm's efficiency and enable you to optimize it if needed.
Coding interview tips
In addition to algorithm problem solving techniques, mastering coding interviews requires a set of specific skills and strategies. Here are some valuable tips to help you navigate through coding interviews and increase your chances of success.
1. Study the fundamentals
Before diving into coding interview preparation, ensure you have a strong foundation in programming fundamentals. Review key concepts like data structures, algorithms, and time complexity analysis. Solidify your understanding of programming languages, including their syntax and standard libraries.
2. Practice coding regularly
The saying "practice makes perfect" holds true when it comes to coding interviews. Regular coding practice helps you improve problem-solving skills and become familiar with common algorithms and data structures. Solve coding problems from various online platforms, participate in coding competitions, and implement side projects to gain hands-on experience.
3. Solve real interview problems
Simulate a real coding interview experience by solving interview-style problems. Online platforms like LeetCode, HackerRank, and CodeSignal provide a wide range of interview-specific coding problems. Familiarize yourself with the types of problems commonly asked in coding interviews, such as array manipulation, string manipulation, sorting, and searching.
4. Master common algorithmic patterns
Many coding problems follow specific algorithmic patterns. By mastering these patterns, you can quickly recognize and solve similar problems. Some common patterns include two pointer technique, sliding window technique, binary search, and dynamic programming. Understand these patterns and practice applying them to different problems.
5. Learn from others
Participating in coding communities and discussion forums can be incredibly helpful. Engage in code review sessions, join coding groups, and learn from experienced programmers. Attend meetups, webinars, or workshops where experts share their insights and techniques for cracking coding interviews.
6. Mock interviews
Mock interviews are an excellent way to simulate the actual coding interview environment. Find a coding buddy or join a mock interview platform to practice solving problems under time pressure. Seek feedback from your mock interviewer and work on areas where you struggle. Continuously refining your interview skills through mock interviews will boost your confidence and performance.
7. Stay calm and communicate
During a coding interview, staying calm and maintaining effective communication with the interviewer is crucial. Start by clarifying any doubts regarding the problem or its requirements. Think out loud and explain your thought process while solving the problem. Properly articulate your approach, code, and optimizations. Effective communication not only demonstrates your technical skills but also enhances your problem-solving capabilities.
Mastering data structures
Data structures are the building blocks of efficient algorithms and form an essential part of cracking coding interviews. Mastering data structures involves understanding their characteristics, operations, and when to use them. Let's explore some fundamental data structures and how to master them.
1. Arrays
Arrays are one of the simplest yet most versatile data structures. Familiarize yourself with array manipulation techniques, such as indexing, appending, and slicing. Learn about multidimensional arrays and their applications. Sharpen your skills in array-related algorithms, such as sorting and searching.
2. Linked Lists
Linked lists provide dynamic memory allocation and efficient insertion and deletion operations. Understand the different types of linked lists, such as singly linked lists, doubly linked lists, and circular linked lists. Learn about their traversal and manipulation techniques. Implement common operations like inserting, deleting, and reversing linked lists.
3. Stacks and Queues
Stacks and queues are abstract data types that follow a specific order of operations. Master their operations, including push, pop, and peek. Understand their applications in solving various coding problems, such as checking balanced parentheses and implementing depth-first and breadth-first traversals.
4. Trees
Trees are hierarchical data structures widely used in coding interview problems. Learn about binary trees, binary search trees, balanced trees, and tries. Understand their traversal techniques, including pre-order, in-order, and post-order traversals. Master tree-related algorithms, such as finding the lowest common ancestor and determining if a binary tree is symmetric.
5. Graphs
Graphs are essential data structures used to represent relationships between entities. Familiarize yourself with different graph representations, such as adjacency matrices and adjacency lists. Learn about graph traversal algorithms like depth-first search and breadth-first search. Understand common graph problems, such as finding the shortest path and detecting cycles.
6. Hash Tables
Hash tables, often implemented as hash maps or dictionaries, offer fast key-value pair lookups. Master hash table operations, such as insertion, deletion, and retrieval. Understand how hashing and collision resolution techniques work. Practice solving coding problems involving hash tables, such as finding duplicate elements and implementing caches.
By thoroughly understanding algorithm problem solving techniques, implementing coding interview tips, and mastering data structures, you can significantly improve your chances of cracking the coding interview. Remember, persistence and regular practice are key to achieving mastery. Good luck with your preparation and ace that coding interview!