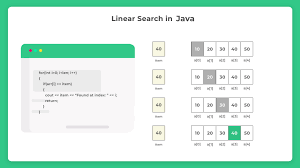
Introduction
Linear search in java, also known as sequential search, is a basic searching algorithm used to find a specific value within a collection of data. It works by sequentially examining each element in the data structure until the target element is found or the entire collection has been traversed. While linear search is relatively simple to implement, it may not be the most efficient algorithm for large datasets, particularly when compared to more advanced search algorithms like binary search.
The process of linear search can be summarized in the following steps:
1. Start from the first element of the data structure (e.g., an array or a list).
2. Compare the current element with the target value that you are searching for.
3. If the current element matches the target value, the search is successful, and the index (position) of the element is returned.
4. If no match is found, move to the next element in the data structure and repeat the comparison process.
5. Continue this process until either the target element is found or the end of the data structure is reached.
6. If the entire data structure has been traversed without finding a match, the search is unsuccessful, and a specific value (such as -1) is often returned to indicate that the target element is not present in the collection.
Linear search is particularly suitable for smaller datasets or when the data is not sorted, as it does not require any specific ordering of elements. However, for larger datasets, more advanced algorithms like binary search or hash-based methods are generally preferred due to their improved efficiency.
One practical example of linear search is looking up a contact in an unsorted list of names. You would start at the beginning of the list and compare each name with the one you are searching for until you find a match or reach the end of the list.
While linear search may not be the fastest algorithm in all cases, understanding its concept is important as it forms the basis for more complex searching and sorting algorithms. It's often taught as an introductory algorithm in programming courses to help learners grasp fundamental concepts before diving into more advanced topics.
Understanding Linear Search in Java
Linear search in java, also known as sequential search, is a straightforward algorithm used to find a particular value within an array. It involves sequentially scanning each element of the array until the desired element is located or the entire array has been traversed. While linear search is not the most efficient algorithm for large datasets, its simplicity and ease of implementation make it a valuable tool for smaller arrays or when the data is not sorted.
The Mechanism of Linear Search or sequential search
Let's delve into the mechanics of linear search or sequential search through a step-by-step process:
1. Initialization: Begin by initializing a loop that iterates through each element of the array. This is typically done using a 'for' loop or a 'while' loop.
2. Comparison: Compare the current element with the target element you are searching for. Use a conditional statement to check if the current element matches the target.
3. Finding the Target: If a match is found, the linear search terminates, and the index of the element is returned. Otherwise, the loop continues to the next element.
4. End of Array: If the loop reaches the end of the array without finding a match, the search concludes, and a specified value (such as -1) is returned to indicate that the target element is not present in the array.
Converting Characters to Strings in Java
Before we dive deeper into the implementation of linear search, let's briefly touch upon the process of converting characters to strings in Java.
In Java, converting a single character to a string can be achieved using various approaches. One common method is to use the `Character.toString(char)` static method, which converts a character to its corresponding string representation. Another approach is to concatenate the character with an empty string, effectively coercing it into a string.
For example:
```java
char myChar = 'A';
String myString = Character.toString(myChar);
// Alternatively:
String myString = myChar + "";
```
Both of these methods yield the same result, providing a string representation of the character.
Implementation of Linear Search
Now, let's delve into the implementation of linear search in Java, incorporating the concepts we've discussed so far:
```java
public class LinearSearch {
public static int linearSearch(int[] array, int target) {
for (int i = 0; i < array.length; i++) {
if (array[i] == target) {
return i; // Return the index of the target element
}
}
return -1; // Target element not found
}
public static void main(String[] args) {
int[] numbers = { 10, 25, 5, 18, 30, 7 };
int target = 18;
int index = linearSearch(numbers, target);
if (index != -1) {
System.out.println("Target element found at index: " + index);
} else {
System.out.println("Target element not found in the array.");
}
}
}
```
In this example, we've defined a method `linearSearch` that accepts an array and a target element as arguments. The method iterates through the array using a 'for' loop, comparing each element with the target. If a match is found, the method returns the index of the target; otherwise, it returns -1.
Scenarios for Using Linear Search
While linear search might not be the most efficient algorithm for large datasets, it finds its usefulness in various scenarios:
1. Small Arrays: Linear search is well-suited for small arrays where the overhead of more complex algorithms might outweigh the benefits.
2. Unsorted Data: When the data is not sorted, linear search remains a viable option since other algorithms might require sorted data.
3. Partial Matches: Linear search can be adapted to search for partial matches, such as substrings within strings.
4. Educational Purposes: Linear search is often taught as an introductory algorithm, helping beginners grasp the concept of searching algorithms before delving into more advanced techniques.
Conclusion
In the realm of programming, understanding the basics is paramount to building a strong foundation. Linear search in java, a simple yet effective algorithm, exemplifies this principle. While not the most efficient choice for all scenarios, linear search finds its value in specific use cases, such as small arrays or unsorted data. By grasping the mechanics of linear search, including concepts like converting characters to strings in Java, developers gain a deeper appreciation for the intricacies of algorithm design.
As we've explored the mechanics, implementation, and scenarios of linear search, we've reiterated essential keywords like 'linear search in Java' and 'char to string Java.' These concepts not only enhance our understanding of linear search but also highlight the interconnected nature of programming concepts.
Incorporating linear search into your programming toolkit provides a valuable tool for data retrieval, and the knowledge gained from understanding its principles can serve as a stepping stone to more advanced algorithms. So, the next time you find yourself faced with a small array or unsorted data, remember the efficiency and simplicity of linear search—it might be just the solution you need.