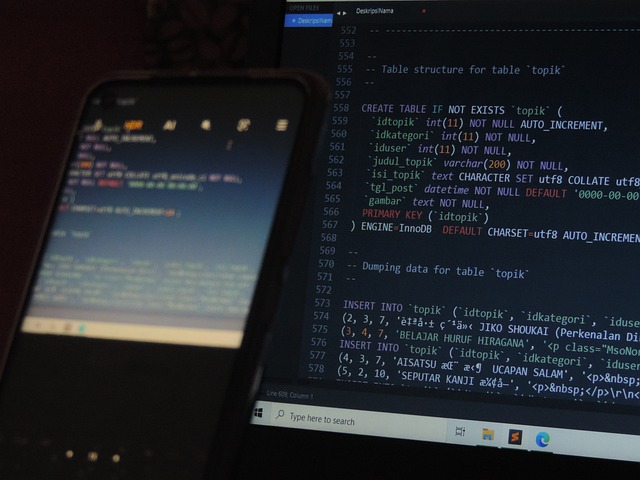
Structured Query Language (SQL) is the cornerstone of database management systems, playing a pivotal role in handling and manipulating data. Whether you're a seasoned database professional or a job seeker aiming to land a position in the realm of data management, SQL proficiency is a must. In this comprehensive guide, we'll delve into the top 40 SQL interview questions and provide detailed answers to help you prepare for your next SQL-related job interview.
Basic SQL Concepts:
1. What is SQL?
Answer: SQL, or Structured Query Language, is a programming language designed for managing and manipulating relational databases. It provides a standardized way to interact with databases, enabling users to create, retrieve, update, and delete data.
2. Differentiate between SQL and MySQL.
Answer: SQL is a language, while MySQL is a relational database management system (RDBMS) that uses SQL. In other words, SQL is the language you use to communicate with databases, and MySQL is one of the database systems that understands and processes SQL queries.
3. Explain the primary types of SQL commands.
Answer:
- Data Definition Language (DDL): Used for defining and managing database structures, including creating, altering, and deleting tables.
- Data Manipulation Language (DML): Involves operations like inserting, updating, and deleting data.
- Data Control Language (DCL): Manages access and permissions to the database, including GRANT and REVOKE statements.
- Transaction Control Language (TCL): Manages transactions within a database, including COMMIT and ROLLBACK.
4. What is a primary key?
Answer: A primary key is a unique identifier for a record in a table. It ensures that each record can be uniquely identified and is used to establish relationships between tables. A primary key cannot have NULL values.
5. Explain the concept of a foreign key.
Answer: A foreign key is a field in a database table that refers to the primary key in another table. It establishes a link between the two tables, creating a relationship. This relationship helps maintain referential integrity and ensures consistency in the data.
SQL Querying:
6. Write a SQL query to retrieve all columns from a table named 'employees.'
Answer:
sql Copy code SELECT * FROM employees;
7. How do you retrieve unique values from a column?
Answer:
sql Copy code SELECT DISTINCT column_name FROM table_name;
8. Explain the difference between INNER JOIN and LEFT JOIN.
Answer:
- INNER JOIN: Returns only the rows where there is a match in both tables.
- LEFT JOIN (or LEFT OUTER JOIN): Returns all rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for columns from the right table.
9. Write a SQL query to find the second-highest salary from a 'salaries' table.
Answer:
sql Copy code SELECT MAX(salary) FROM salaries WHERE salary < (SELECT MAX(salary) FROM salaries);
10. Explain the purpose of the GROUP BY clause.
Answer: The GROUP BY clause is used to group rows based on the values in one or more columns. It is often used with aggregate functions like COUNT, SUM, AVG, etc., to perform operations on each group of rows.
Advanced SQL Concepts:
11. What is a stored procedure?
Answer: A stored procedure is a precompiled collection of one or more SQL statements that can be executed as a single unit. It is stored in the database and can be called and executed multiple times.
12. Differentiate between UNION and UNION ALL.
Answer:
- UNION: Combines and returns distinct rows from the result sets of two or more SELECT statements.
- UNION ALL: Combines and returns all rows, including duplicates, from the result sets of two or more SELECT statements.
13. Explain the ACID properties of a transaction.
Answer:
- Atomicity: Ensures that a transaction is treated as a single, indivisible unit. Either all its changes are committed, or none are.
- Consistency: Ensures that a transaction brings the database from one valid state to another. All integrity constraints are maintained.
- Isolation: Ensures that the execution of one transaction is isolated from the execution of other transactions.
- Durability: Once a transaction is committed, its changes are permanent and survive future system failures.
14. How do you optimize a SQL query?
Answer:
- Use indexes: Indexes can significantly speed up data retrieval.
- *Limit the use of SELECT : Only retrieve the columns you need.
- Optimize WHERE clauses: Use appropriate operators and conditions.
- Avoid using too many JOINs: Excessive joins can impact performance.
15. What is normalization, and why is it important?
Answer: Normalization is the process of organizing data in a database to reduce redundancy and dependency. It involves dividing large tables into smaller, more manageable tables and defining relationships between them. Normalization helps improve data integrity and reduces the chances of data anomalies.
SQL Security and Optimization:
16. Explain SQL injection.
Answer: SQL injection is a type of cyberattack where an attacker injects malicious SQL code into a query to manipulate the database. It often occurs when user inputs are not properly validated or sanitized, allowing unauthorized access to the database.
17. How can you prevent SQL injection?
Answer:
- Use parameterized queries: Instead of concatenating values into SQL statements, use parameters.
- Input validation: Ensure that user inputs meet expected criteria.
- Use stored procedures: They can help mitigate the risk of SQL injection.
18. What is indexing, and why is it important for performance?
Answer: Indexing is the process of creating a data structure to improve the speed of data retrieval operations on a database table. Indexes provide a quick lookup mechanism, significantly reducing the time it takes to fetch specific rows. Properly indexed tables can dramatically enhance query performance.
19. Explain the purpose of the EXPLAIN statement.
Answer: The EXPLAIN statement is used to analyze and optimize the execution plan of a SQL query. It provides information about how the database engine will execute the query, including details about the order of table access, use of indexes, and the overall execution strategy.
20. How can you monitor and optimize database performance?
Answer:
- Regularly analyze query performance: Identify slow-performing queries and optimize them.
- Use database monitoring tools: Monitor resource usage, query execution times, and other relevant metrics.
- Optimize indexes: Ensure that tables are properly indexed for efficient data retrieval.
Data Modification and Transactions:
21. Explain the purpose of the COMMIT statement.
Answer: The COMMIT statement is used to save all changes made during the current transaction to the database. Once a COMMIT statement is executed, the changes become permanent.
22. What is a rollback in SQL?
Answer: A rollback is a SQL operation that undoes the changes made during the current transaction. It is used to revert the database to its state before the transaction began, effectively canceling the transaction.
23. How do you add a new record to a table?
Answer:
sql Copy code INSERT INTO table_name (column1, column2, column3, ...) VALUES (value1, value2, value3, ...);
24. Explain the purpose of the UPDATE statement.
Answer: The UPDATE statement is used to modify existing records in a table. It allows you to change the values of specific columns based on specified conditions.
25. What is the purpose of the DELETE statement?
Answer: The DELETE statement is used to remove one or more records from a table based on specified conditions. It permanently deletes data from the table.
Advanced Querying:
26. How can you find the Nth highest or lowest value in a column?
Answer:
To find the Nth highest value:
sql Copy code SELECT DISTINCT column_name FROM table_name ORDER BY column_name DESC LIMIT 1 OFFSET (N - 1);
To find the Nth lowest value:
sql Copy code SELECT DISTINCT column_name FROM table_name ORDER BY column_name ASC LIMIT 1 OFFSET (N - 1);
27. Explain the HAVING clause.
Answer: The HAVING clause is used in combination with the GROUP BY clause to filter the results of aggregate functions. It allows you to specify conditions on the aggregated data.
28. Write a SQL query to get the total number of rows in a table.
Answer:
sql Copy code SELECT COUNT(*) FROM table_name;
29. How do you perform a case-insensitive search in SQL?
Answer:
sql Copy code SELECT * FROM table_name WHERE LOWER(column_name) = LOWER('search_value');
30. Explain the difference between a subquery and a JOIN.
Answer:
- Subquery: A subquery is a query embedded within another query. It is enclosed in parentheses and executed independently within the main query.
- JOIN: JOIN is used to combine rows from two or more tables based on a related column between them. It retrieves columns from both tables in the result set.
Transactions and Isolation Levels:
31. What is a transaction in SQL?
Answer: A transaction in SQL is a sequence of one or more SQL statements that are executed as a single unit of work. Transactions ensure data consistency and integrity by either committing all changes or rolling back to the previous state if an error occurs.
32. Explain the concept of isolation levels in SQL.
Answer: Isolation levels define the degree to which one transaction is isolated from the effects of other concurrent transactions. The four standard isolation levels are READ UNCOMMITTED, READ COMMITTED, REPEATABLE READ, and SERIALIZABLE, each offering a different level of consistency and concurrency.
33. How does the ACID property relate to transactions?
Answer: The ACID properties (Atomicity, Consistency, Isolation, and Durability) ensure the reliability and integrity of transactions. These properties guarantee that database transactions are processed reliably even in the event of system failures or errors.
34. What is a savepoint in SQL?
Answer: A savepoint is a point within a transaction to which you can later roll back. It allows you to create a partial rollback within a transaction, reverting changes up to a specific savepoint without rolling back the entire transaction.
35. Explain the purpose of the ROLLBACK TO SAVEPOINT statement.
Answer: The ROLLBACK TO SAVEPOINT statement is used to undo transactions up to a specified savepoint within a transaction. It allows for partial rollback, reverting changes made after a specific savepoint without affecting the entire transaction.
Performance Tuning and Optimization:
36. How can you improve the performance of a SQL query?
Answer:
- Use appropriate indexes: Indexes can significantly speed up data retrieval.
- Optimize WHERE clauses: Use efficient conditions and operators.
- *Limit the use of SELECT : Only retrieve the columns you need.
- Analyze and optimize the execution plan: Use the EXPLAIN statement to understand and optimize the query plan.
37. What is database normalization, and why is it essential for performance?
Answer: Database normalization is the process of organizing data in a database to reduce redundancy and dependency. While normalization is crucial for data integrity, it may have a trade-off in terms of performance. Over-normalization can lead to complex JOIN operations, impacting query performance. Striking a balance between normalization and performance is essential.
38. Explain the concept of query optimization.
Answer: Query optimization is the process of improving the performance of a query by selecting the most efficient execution plan. This involves optimizing the structure of the query, indexing tables appropriately, and analyzing the query execution plan to identify bottlenecks and areas for improvement.
39. What is an execution plan in SQL, and how can you view it?
Answer: An execution plan is a detailed blueprint that the database engine uses to execute a query. It shows the steps the database engine takes to retrieve and process the data. You can view the execution plan using the EXPLAIN statement or through database management tools.
40. How can you handle large datasets in SQL?
Answer:
- Use pagination: Retrieve and display data in smaller chunks using the LIMIT and OFFSET clauses.
- Optimize queries: Use appropriate indexes and optimize the execution plan.
- Consider partitioning: Partition large tables to improve query performance.
Conclusion:
Mastering SQL is essential for anyone working with databases, and proficiency in SQL is a key requirement in many job interviews. This guide covers a diverse range of SQL topics, from fundamental concepts to advanced querying, security, transactions, and performance optimization. By familiarizing yourself with these top 40 SQL interview questions and answers, you'll be well-prepared to showcase your SQL skills and excel in your next database-related job interview. Remember to practice these concepts in real-world scenarios and explore hands-on examples to solidify your understanding of SQL.
Also Check out!
- Simplilearn
- Simpliearn Data Science Course Review
- Simplilearn Review
- Imarticus Data Science Course Review
- Imarticus
- Great Learning Reviews
- UpGrad Reviews