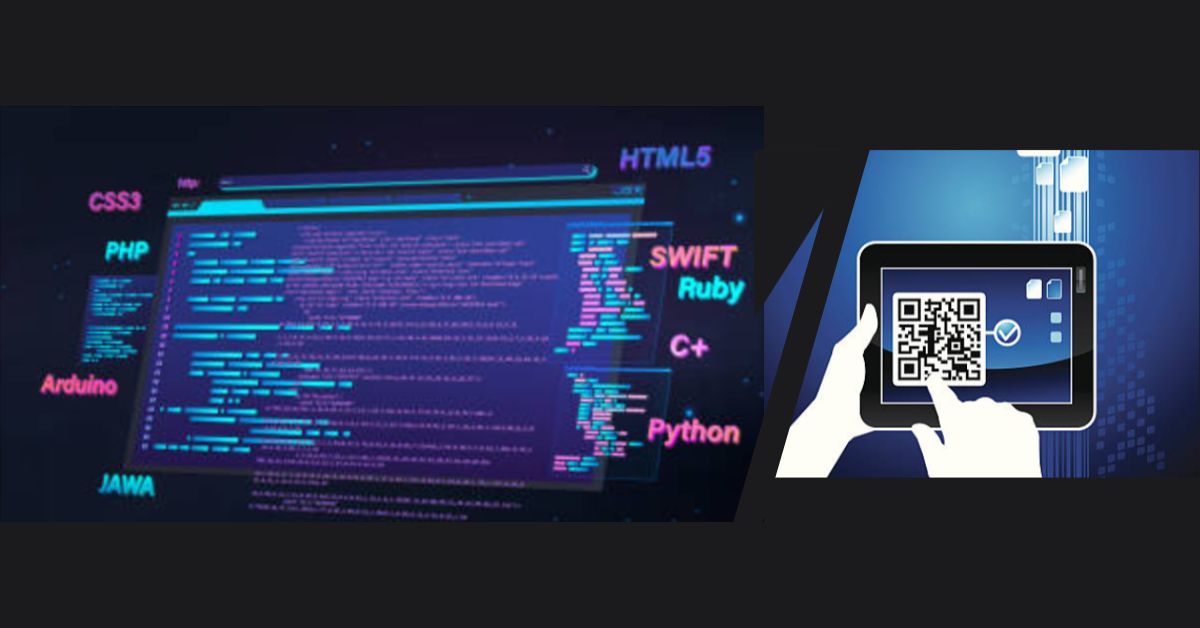
To implement barcode and QR code scanning in your iOS and Android native apps using Dynamsoft Barcode Reader, you'll need to follow these general steps. Keep in mind that you should refer to the official documentation for the most accurate and up-to-date information:
1. Obtain Dynamsoft Barcode Reader SDK:
- Visit Dynamsoft for barcode reader demo
- Download the SDK for iOS and Android.
2. Set Up Your Development Environment:
For iOS:
- Open your Xcode project.
- Drag and drop the DynamsoftBarcodeReader.framework into your project.
- Add the necessary permissions to your app's Info.plist file.
For Android:
- Open your Android Studio project.
- Add the Dynamsoft Barcode Reader library to your project.
3. Initialize Dynamsoft Barcode Reader:
For iOS:
swift Copy code import DynamsoftBarcodeReader // Initialize Dynamsoft Barcode Reader let barcodeReader = DynamsoftBarcodeReader() // Set license key (replace YOUR_LICENSE_KEY with your actual license key) barcodeReader.initLicense("YOUR_LICENSE_KEY")
For Android:
java Copy code import com.dynamsoft.barcode.BarcodeScanner; // Initialize Dynamsoft Barcode Reader BarcodeScanner barcodeScanner = new BarcodeScanner(); // Set license key (replace YOUR_LICENSE_KEY with your actual license key) barcodeScanner.initLicense("YOUR_LICENSE_KEY");
4. Implement Barcode Scanning:
For iOS:
swift Copy code // Implement barcode scanning let results = barcodeReader.decodeFile("path/to/your/image.jpg") for result in results { print("Barcode Format: \(result.barcodeFormat), Value: \(result.barcodeText)") }
For Android:
java Copy code // Implement barcode scanning String[] results = barcodeScanner.decodeFile("path/to/your/image.jpg"); for (String result : results) { System.out.println("Barcode Value: " + result); }
5. Integrate QR Code Scanning:
The steps for QR code scanning are similar to barcode scanning, as Dynamsoft Barcode Reader supports both.
6. Handle Permissions:
Ensure that your app has the necessary permissions to access the camera for barcode/QR code scanning.
7. UI Integration:
Create a user interface that allows users to trigger the barcode/QR code scanning process.
8. Testing:
Test your app on both iOS and Android devices to ensure the barcode and QR code scanning functionality works as expected.
9. Error Handling and Optimization:
Implement proper error handling and optimize your code for performance.