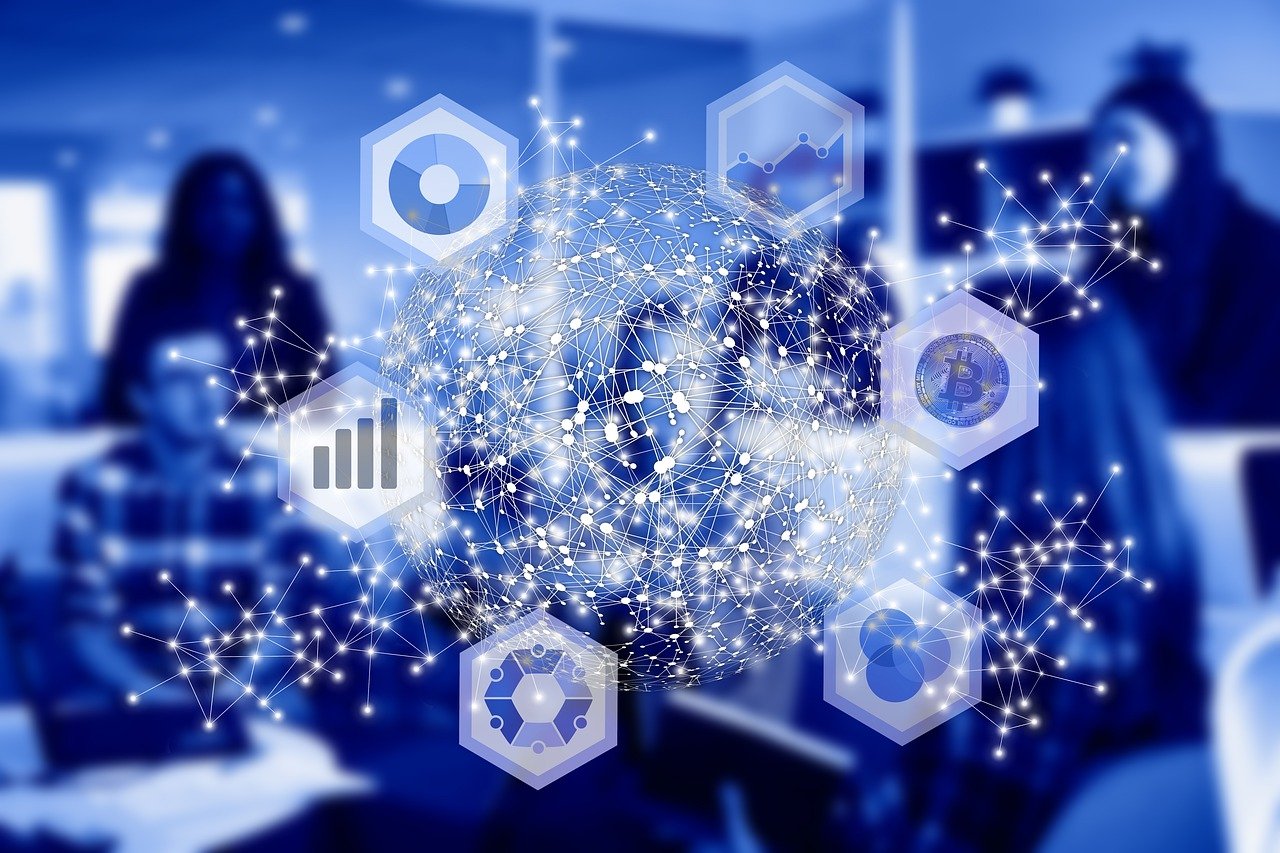
In the realm of data analysis and statistics, understanding the deviation and distribution of data is crucial for making informed decisions, drawing meaningful insights, and building robust models. Python, with its extensive libraries like NumPy, SciPy, and Matplotlib, offers powerful tools for exploring, visualizing, and analyzing data distributions.
Explore deep into the concepts of data deviation and distribution, and how to effectively leverage Python for insightful analysis.
Understanding Data Deviation:
Data deviation, also known as variance, measures the spread or dispersion of a dataset around its mean. It provides valuable information about how data points are dispersed from the central tendency. The standard deviation, a widely used metric for deviation, quantifies the average distance of data points from the mean.
In Python, calculating the deviation of a dataset is straightforward using libraries like NumPy. The `numpy.var()` function computes the variance, while `numpy.std()` calculates the standard deviation.
```python
import numpy as np
data = np.array([5, 7, 8, 10, 12, 15])
variance = np.var(data)
std_deviation = np.std(data)
print("Variance:", variance)
print("Standard Deviation:", std_deviation)
```
Understanding Data Distribution:
Data distribution describes the way data is spread across various values in a dataset. It provides insights into the probability of different outcomes and forms the basis for many statistical analyses. Common types of distributions include normal (Gaussian), binomial, uniform, and exponential distributions.
Python offers powerful tools for visualizing and analyzing data distributions. Matplotlib, seaborn, and scipy.stats are popular libraries for this purpose. Let's explore how to create histograms, density plots, and cumulative distribution functions (CDFs) using Matplotlib and scipy.stats.
```python
import matplotlib.pyplot as plt
import seaborn as sns
from scipy.stats import norm
Also Check:
· best data science course in delhi
· best institutes for data science course in delhi
· top institutes for data science course in delhi
· best data science course in delhi with placement guarantee
# Generate random data from a normal distribution
data = np.random.normal(loc=0, scale=1, size=1000)
# Plot histogram
plt.figure(figsize=(10, 6))
sns.histplot(data, kde=True, bins=30, color='skyblue')
plt.title('Histogram of Data Distribution')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
# Plot density plot
plt.figure(figsize=(10, 6))
sns.kdeplot(data, color='red', shade=True)
plt.title('Density Plot of Data Distribution')
plt.xlabel('Value')
plt.ylabel('Density')
plt.show()
# Plot cumulative distribution function (CDF)
plt.figure(figsize=(10, 6))
sns.ecdfplot(data)
plt.title('Cumulative Distribution Function (CDF)')
plt.xlabel('Value')
plt.ylabel('Cumulative Probability')
plt.show()
```
Analyzing Data Deviation and Distribution:
Once we have a grasp of data deviation and distribution, we can perform various analyses to gain insights into the dataset. For instance, we can identify outliers, assess the normality of the distribution, or compare different datasets.
To identify outliers, we can use methods such as Z-score or IQR (Interquartile Range). Python provides convenient functions and libraries to implement these methods.
```python
# Detect outliers using Z-score
z_scores = (data - np.mean(data)) / np.std(data)
outliers = np.where(np.abs(z_scores) > 3)[0]
print("Outliers using Z-score:", outliers)
# Detect outliers using IQR
Q1 = np.percentile(data, 25)
Q3 = np.percentile(data, 75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
outliers_iqr = np.where((data < lower_bound) | (data > upper_bound))[0]
print("Outliers using IQR:", outliers_iqr)
```
Conclusion:
In this guide, we've explored the fundamental concepts of data deviation and distribution, and how Python can be utilized for in-depth analysis. By leveraging Python libraries such as NumPy, Matplotlib, and scipy.stats, we can efficiently calculate deviations, visualize distributions, and perform advanced statistical analyses.