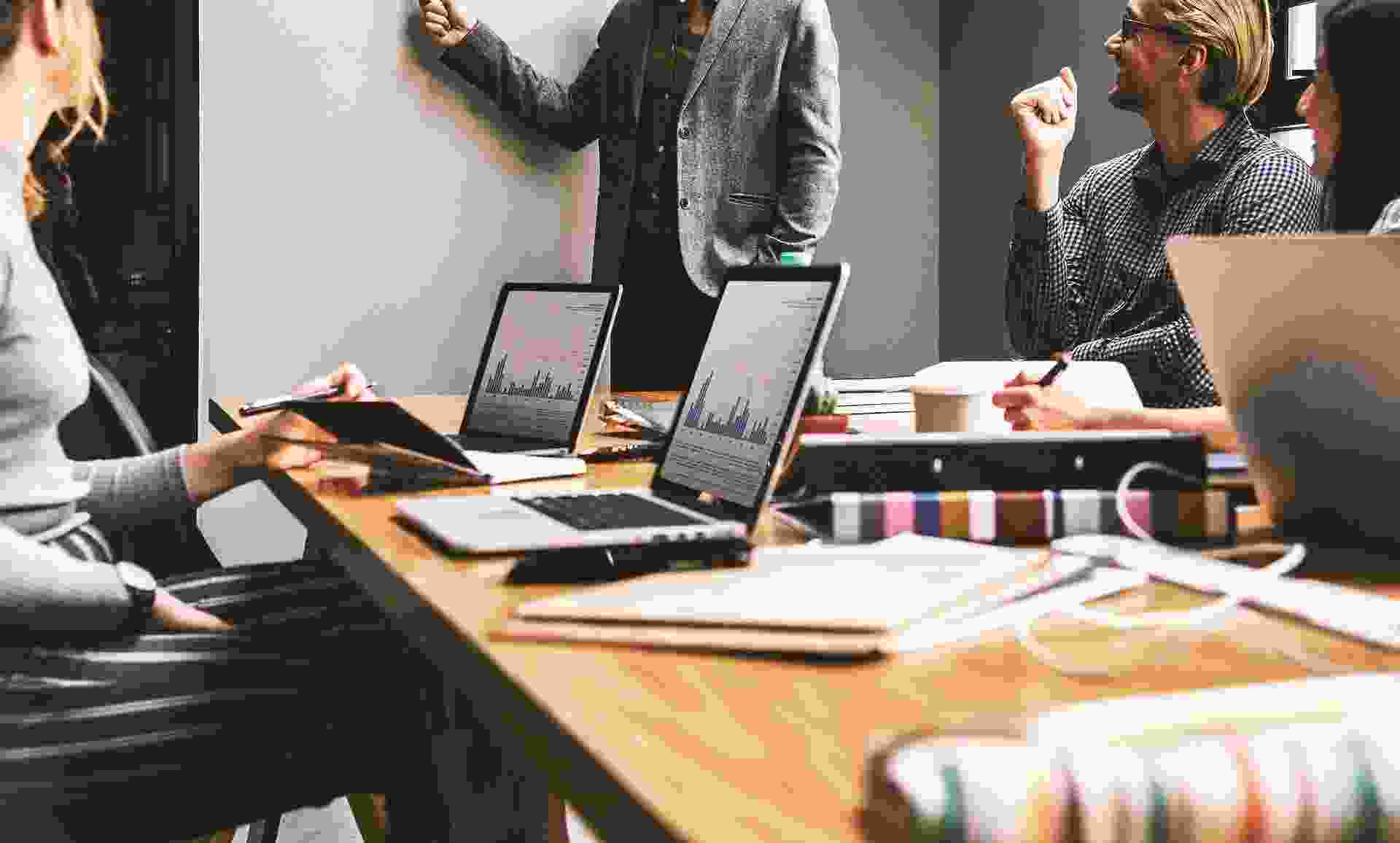
Probability theory is a fundamental concept in mathematics and statistics that plays a crucial role in various fields such as data science, machine learning, and artificial intelligence. In Python, there are several libraries and methods available to work with different types of probability distributions and calculations. In this comprehensive guide, we will explore the different types of probability used in Python, including theoretical probability, empirical probability, conditional probability, and Bayesian probability, along with practical examples and implementations.
Theoretical Probability:
Theoretical probability, also known as classical probability, is based on the assumption of equally likely outcomes. It involves calculating the probability of an event by dividing the number of favorable outcomes by the total number of possible outcomes. In Python, you can calculate theoretical probability using simple arithmetic operations.
```python
# Example of theoretical probability calculation
def theoretical_probability(event_outcomes, sample_space):
return event_outcomes / sample_space
# Calculate the probability of rolling a 4 on a fair six-sided die
event_outcomes = 1 # There is one favorable outcome (rolling a 4)
sample_space = 6 # Total number of possible outcomes (six-sided die)
probability = theoretical_probability(event_outcomes, sample_space)
print("Theoretical Probability:", probability)
```
Empirical Probability:
Empirical probability is based on observed frequencies from data. It involves calculating the probability of an event by analyzing historical or experimental data. In Python, you can compute empirical probability using frequency counts or by analyzing datasets.
```python
# Example of empirical probability calculation using frequency counts
def empirical_probability(event_count, total_trials):
return event_count / total_trials
# Suppose we conduct an experiment of flipping a coin 100 times
# and observe that it lands on heads 55 times
event_count = 55 # Number of times the event occurred (heads)
total_trials = 100 # Total number of trials (coin flips)
probability = empirical_probability(event_count, total_trials)
print("Empirical Probability:", probability)
```
Conditional Probability:
Conditional probability is the probability of an event occurring given that another event has already occurred. It is calculated by dividing the joint probability of both events by the probability of the given event. In Python, you can compute conditional probability using conditional statements and probability rules.
```python
# Example of conditional probability calculation
def conditional_probability(event_a_count, event_b_count, total_trials):
return (event_a_count / total_trials) / (event_b_count / total_trials)
# Suppose we have data on the occurrence of two events A and B
# and we want to calculate the probability of event A given that event B has occurred
event_a_count = 30 # Number of times event A occurred
event_b_count = 50 # Number of times event B occurred
total_trials = 1000 # Total number of trials
probability = conditional_probability(event_a_count, event_b_count, total_trials)
print("Conditional Probability of A given B:", probability)
```
Bayesian Probability:
Bayesian probability is a statistical method that represents uncertainty using probability distributions. It involves updating prior beliefs based on observed evidence to obtain posterior probabilities. In Python, you can perform Bayesian inference using libraries such as PyMC3 or Stan.
```python
# Example of Bayesian probability calculation using PyMC3
import pymc3 as pm
# Define prior distribution
prior = pm.Beta('prior', alpha=1, beta=1)
# Define likelihood function
likelihood = pm.Binomial('likelihood', n=10, p=prior, observed=7)
# Perform Bayesian inference
with pm.Model() as model:
trace = pm.sample(1000, tune=1000)
# Plot posterior distribution
pm.plot_posterior(trace)